Tech Tip: Utility method to get distinct values and its number of occurrences in an array
PRODUCT: 4D | VERSION: 13.2 | PLATFORM: Mac & Win
Published On: March 5, 2013
This Technical Tip provides a utility method that takes in an array and will return a two-dimensional array populated with distinct values along with the count for the number of occurrences for each distinct value.
// ---------------------------------------------------- // Method: UTIL_ARRAY_COUNT_VALUES // Description // Utility method used to get distinct values of an array along with // the number of occurrences of each distinct value // // Parameters // $1 (Pointer) - Pointer to a 1-dimensional text array // $2 (Pointer) - Pointer to a 2-dimensional text array where the distinct values // and the count for the number of occurrences will be stored // ---------------------------------------------------- C_POINTER($1;$array_ptr) C_POINTER($2;$distinctVals_ptr) C_LONGINT($arrSize_l) C_LONGINT($i;$pos) $array_ptr:=$1 $distinctVals_ptr:=$2 $arrSize_l:=Size of array($array_ptr->) $i:=1 While ($i<=$arrSize_l) //check to see if we have already encountered this value $pos:=Find in array($distinctVals_ptr->{1};$array_ptr->{$i}) //if the value is not already stored in the distinct values array, add it If ($pos=-1) APPEND TO ARRAY($distinctVals_ptr->{1};$array_ptr->{$i}) APPEND TO ARRAY($distinctVals_ptr->{2};String(1)) //if the value already exists in the distinct values array, //increase the count for the number of occurrences Else $distinctVals_ptr->{2}{$pos}:=String(Num($distinctVals_ptr->{2}{$pos})+1) End if $i:=$i+1 End while |
Here is an example method that calls UTIL_ARRAY_COUNT_VALUES:
ARRAY TEXT(myVals_at;0) ARRAY TEXT(distinctVals_2Dat;2;0) APPEND TO ARRAY(myVals_at;"A") APPEND TO ARRAY(myVals_at;"B") APPEND TO ARRAY(myVals_at;"A") APPEND TO ARRAY(myVals_at;"C") APPEND TO ARRAY(myVals_at;"C") APPEND TO ARRAY(myVals_at;"A") APPEND TO ARRAY(myVals_at;"D") UTIL_ARRAY_COUNT_VALUES (->myVals_at;->distinctVals_2Dat) |
To better visualize this example, here are what the two arrays look like once the above code is executed:
Array myVals_at:

Array distinctVals_2Dat:
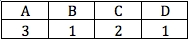