Tech Tip: Extracting the field names and corresponding types from a table
PRODUCT: 4D | VERSION: 15 | PLATFORM: Mac & Win
Published On: October 21, 2015
Similar to Tech Tip #77302 "Getting a list of field names from a table name", here is a method that will extract a field name and the type to an array. This can bring extra redundancy on checking the data type entry of a field before saving the record:
// --------------------------------------------------------------------------- // Name: GetFieldTypesFromTable // Description: Method will take in a pointer to a Table and update a // pointer to an array of the Field and Field type of the Table. // // Parameters: // $1 (POINTER) - Pointer to the 4D Table to reference. // $2 (POINTER) - Pointer to an array to return the Field name (Text). // $3 (POINTER) - Pointer to an array to return the Field type (LongInt). // --------------------------------------------------------------------------- C_POINTER($1;$table) C_POINTER($2;$arrFieldName) C_POINTER($3;$arrFieldType) C_POINTER($field_ptr) C_LONGINT($i) If (Count parameters>=3) $table:=$1 $arrFieldName:=$2 $arrFieldType:=$3 For ($i;1;Get last field number($table)) $field_ptr:=Field(Table($table);$i) APPEND TO ARRAY($arrFieldName->;Field name(Table($table);$i)) // Field Name (Text) APPEND TO ARRAY($arrFieldType->;Type($field_ptr->)) // Field Type (Longint) End for End if |
Here is example of a Table and using the method GetFieldTypesFromTable:
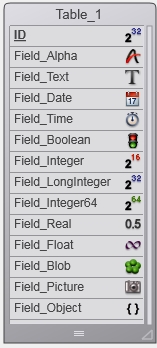
ARRAY TEXT(arrFieldName;0) ARRAY LONGINT(arrFieldType;0) GetFieldTypesFromTable (->[Table_1];->arrFieldName;->arrFieldType) |
The arrays are filled with the following:
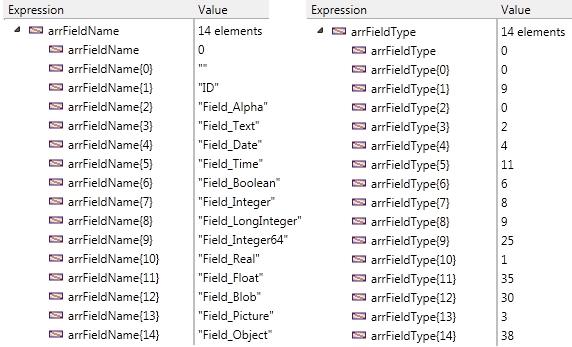
The array arrFieldType represents the constants returned from the Type command.
Here is an example of checking entry validity of a field:
C_TEXT($data_entering) $data_entering:="entering_text" If (Type($data_entering)=arrFieldType{3}) // Text type [Table_1]Field_Text:=$data_entering End if |
See Also: