Tech Tip: Creating a status bar in a List Box
PRODUCT: 4D | VERSION: 14.3 | PLATFORM: Mac & Win
Published On: March 13, 2015
One way to bring pop to the data in a List Box is by incorporating a status bar. Like the progress bar object in a form, the status bar can display a meter like indicator with a percentage. The following methods are needed to implement in a List Box:
// ---------------------------------------------------------------------- // Name: UTIL_DecToHex // Description: Takes in the integer and converts the value into hex. // Parameters: // $1 (LONGINT) - Number to convert to HEX // Output: // $0 (TEXT) - Hex representation // ---------------------------------------------------------------------- C_TEXT($0) C_LONGINT($1) If (Count parameters=1) $0:=Substring(String($1;"&$");2) End if |
LISTBOX_STATUS_BAR method to create the status bar on a List Box:
// ---------------------------------------------------------------------- // Name: LISTBOX_STATUS_BAR // Description: Draws a status bar with a percentage in SVG. // Will get the List Box column and width dimensions to draw the // status bar with a random gradient color effect and percentage. // Parameters: // $1 (TEXT) - List Box object name height // $2 (TEXT) - List Box column name for column width // $3 (LONGINT) - Percent count to display the progress status 1-100% // Output: // $0 (PICTURE) - Picture with progress and percentage // ---------------------------------------------------------------------- C_TEXT($1) // List Box Object C_TEXT($2) // List Box Column Object C_TEXT($Color1;$Color2) // Colors for gradient C_LONGINT($3) // Count to display C_PICTURE($0;$generated_picure) // Output of the status bar C_LONGINT($height;$length;$posx1;$posx2;$posy1;$posy2) C_REAL($value) // Percentage status $height:=LISTBOX Get rows height(*;$1;Listbox pixels) $length:=LISTBOX Get column width(*;$2;$minWidth;$maxWidth)+1 vID_SVG:=SVG_New($length;$height) $posx1:=0 $posx2:=(($3*$length)/100) $posy1:=0 $posy2:=0 $value:=round($3;0) // Showing the percentage // Random color for gradient $Color1:="#"+UTIL_DecToHex((Random%239)+16)+UTIL_DecToHex ((Random%239)+16)+UTIL_DecToHex ((Random%239)+16) $Color2:="#"+UTIL_DecToHex ((Random%239)+16)+UTIL_DecToHex ((Random%239)+16)+UTIL_DecToHex ((Random%239)+16) SVG_Define_linear_gradient(vID_SVG;"gradient";$Color1;$Color2) // Set the gradient // Draw the status bar $idLine:=SVG_New_line(vID_SVG;$posx1;$posy1;$posx2;$posy2;"gradient";$height*1.9) // Show percentage $idText:=SVG_NEW_text(vID_SVG;String($value)+"%";$length/2.1;$height/3;"";-1;-1;-1;"Black") SVG EXPORT TO PICTURE(vID_SVG;$generated_picure;Copy XML data source) $0:=$generated_picure |
Here is an example of 4 variables that will be incorporated in the List Box:
C_LONGINT($percentage1;$percentage2;$percentage3;$percentage4) ARRAY PICTURE(Column1;4) $percentage1:=15 $percentage2:=78 $percentage3:=44 $percentage4:=2 Column1{1}:=LISTBOX_STATUS_BAR ("List Box";"Column1";$percentage1) Column1{2}:=LISTBOX_STATUS_BAR ("List Box";"Column1";$percentage2) Column1{3}:=LISTBOX_STATUS_BAR ("List Box";"Column1";$percentage3) Column1{4}:=LISTBOX_STATUS_BAR ("List Box";"Column1";$percentage4) |
List Box with percentages:
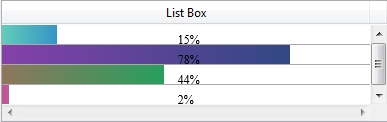
List Box with an adjusted row height:
LISTBOX SET ROWS HEIGHT(*;"List Box";30) |
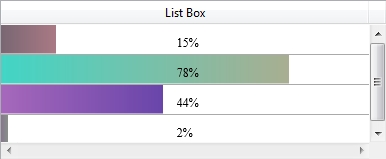
List Box with an increased column width:
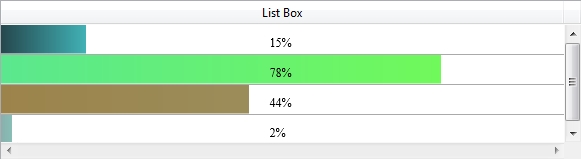
List Box with an even more adjust row height:
LISTBOX SET ROWS HEIGHT(*;"List Box";60) |
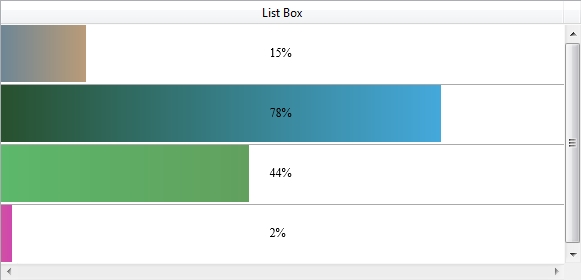
See Also: