Tech Tip: Sorting Roman Numeral Numbers
PRODUCT: 4D | VERSION: 14.3 | PLATFORM: Mac & Win
Published On: April 20, 2015
Below is a method that will sort a text array of Roman Numeral numbers assuming that the values are valid and only Roman Numeral Characters. This method uses the RomToNum method which is described in the "Methods for Roman Numeral Conversions" Tech Tip.
//Method: Roman Sort C_POINTER($1) ARRAY TEXT($arr_at;0) C_POINTER($2) ARRAY LONGINT($arr_al;0) C_LONGINT($temp_l;$i;$j) C_TEXT($temp_t) If (Count parameters=2) COPY ARRAY($1->;$arr_at) For ($i;1;Size of array($arr_at)) APPEND TO ARRAY($arr_al;RomToNum ($arr_at{$i})) End for For ($i;2;Size of array($arr_at)) $temp_l:=($arr_al{$i}) $temp_t:=($arr_at{$i}) $j:=$i While (($j>0) & ($arr_al{$j-1}>$temp_l)) $arr_at{$j}:=$arr_at{$j-1} $arr_al{$j}:=$arr_al{$j-1} $j:=$j-1 $arr_at{$j}:=$temp_t $arr_al{$j}:=$temp_l End while End for COPY ARRAY($arr_at;$2->) End if |
Below is an example of how to use the method:
ARRAY TEXT($arr_at;0) ARRAY TEXT($result_at;0) APPEND TO ARRAY($arr_at;"V") APPEND TO ARRAY($arr_at;"I") APPEND TO ARRAY($arr_at;"II") APPEND TO ARRAY($arr_at;"IV") APPEND TO ARRAY($arr_at;"III") APPEND TO ARRAY($arr_at;"X") APPEND TO ARRAY($arr_at;"VII") APPEND TO ARRAY($arr_at;"I") APPEND TO ARRAY($arr_at;"IX") RomanSort (->$arr_at;->$result_at) |
The results are:
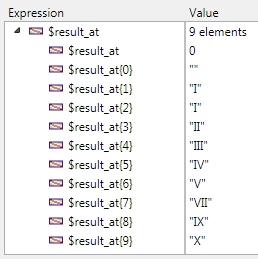
Commented by Lindsay Simpson on April 21, 2015 at 8:20 PM
Couldn't this be done more optimally using SORT ARRAY?
See example below.
//Method: Roman Sort
C_POINTER($1;$2)
C_LONGINT($i)
If (Count parameters>1)
ARRAY TEXT($arr_at;0)
ARRAY LONGINT($arr_al;0)
COPY ARRAY($1->;$arr_at)
For ($i;1;Size of array($arr_at))
APPEND TO ARRAY($arr_al;RomToNum ($arr_at{$i}))
End for
SORT ARRAY($arr_al;$arr_at;>)
COPY ARRAY($arr_at;$2->)
End if