Tech Tip: Utility Method to Modify the Colors of Graph
PRODUCT: 4D | VERSION: 14.3 | PLATFORM: Mac & Win
Published On: May 27, 2015
Below is a method that will allow the colors of a graph created from the GRAPH command to be selected.
//---------------------------------------------------------------- // Method: GRAPH_SET_COLOR // // Description: Changes the colors of a graph created from the GRAPH // command by passing a text array of colors in SVG format // Pass a null in the Text array to keep the data's current color // // Parameters: // $1 - Pointer to the Picture containing the Graph // $2 - Pointer to the Text Array of colors // //---------------------------------------------------------------- C_POINTER($1;$2) C_PICTURE($picture_p) ARRAY TEXT($colors_at;0) C_TEXT($SVGref_t;$xmlElement_t) C_LONGINT($counter_l) If (Count parameters=2) $picture_p:=$1-> COPY ARRAY($2->;$colors_at) $SVGref_t:=SVG_Open_picture ($picture_p) For ($counter_l;1;Size of array($colors_at)) If (($colors_at{$counter_l})#"") $xmlElement_t:=DOM Find XML element by ID($SVGref_t;"ID_graph_"+String($counter_l)) If (OK=1) DOM SET XML ATTRIBUTE($xmlElement_t;"fill";$colors_at{$counter_l}) DOM SET XML ATTRIBUTE($xmlElement_t;"stroke";$colors_at{$counter_l}) End if $xmlElement_t:=DOM Find XML element by ID($SVGref_t;"ID_bullet_"+String($counter_l)) If (OK=1) DOM SET XML ATTRIBUTE($xmlElement_t;"fill";$colors_at{$counter_l}) End if $xmlElement_t:=DOM Find XML element by ID($SVGref_t;"ID_legend_"+String($counter_l)) If (OK=1) DOM SET XML ATTRIBUTE($xmlElement_t;"fill";$colors_at{$counter_l}) End if End if End for SVG EXPORT TO PICTURE($SVGref_t;$picture_p;Copy XML data source) DOM CLOSE XML($SVGref_t) $1->:=$picture_p End if |
Following the style of using the GRAPH command, to use the method an array of colors following the ordering of the data is created and passed along with the resulting 4D picture created with GRAPH.
The text array of colors can be any of the following valid RGB formats also explained in the documentation for SVG colors at:
http://doc.4d.com/4Dv14R5/4D/14-R5/SVG-Colors.300-1889656.en.html
RGB format
Format Example
#rgb #f00
#rrggbb #ff0000
rgb(r,g,b) rgb(255,0,0)
rgb(100%, 0%, 0%)
Example
Below is an example of a GRAPH with default coloring:
C_PICTURE($picture_p) //Variable of graph ARRAY TEXT(X;3) //Create an array for the x-axis X{1}:="Set 1" //X Label #1 X{2}:="Set 2" //X Label #2 X{3}:="Set 3" //X Label #2 ARRAY REAL(A;3) //Create an array for the y-axis A{1}:=30 //Insert some data A{2}:=20 A{3}:=50 ARRAY REAL(B;3) //Create an array for the y-axis B{1}:=50 //Insert some data B{2}:=80 B{3}:=100 ARRAY REAL(C;3) //Create an array for the y-axis C{1}:=10 //Insert some data C{2}:=50 C{3}:=20 vType:=3 //Initialize graph type GRAPH($picture_p;vType;X;A;B;C) //Draw the graph GRAPH SETTINGS($picture_p;0;0;0;0;False;False;True;"Data 1";"Data 2";"Data 3") |
$picture_p is
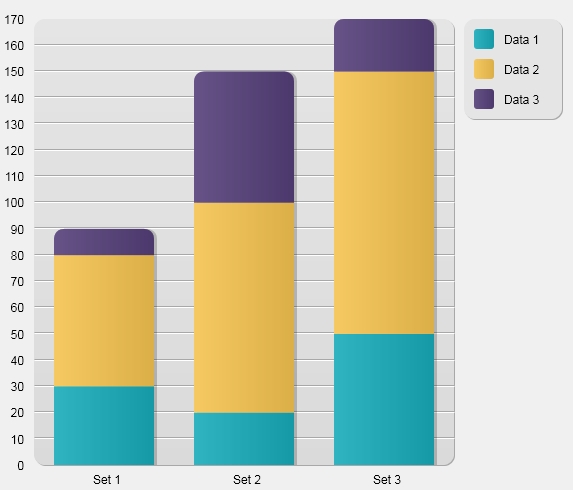
To use the method take the resulting picture variable containing the graph and create an array of colors and pass it into the method:
//Added to the code earlier ARRAY TEXT($color_at;0) //Build a text array with any of the valid RGB formats APPEND TO ARRAY($color_at;"#FF0000")//Red APPEND TO ARRAY($color_at;"rgb(0%,0%,100%)")//Blue APPEND TO ARRAY($color_at;"rgb(0,255,0)")//Green GRAPH_SET_COLOR (->$picture_p;->$color) |
$picture_p is
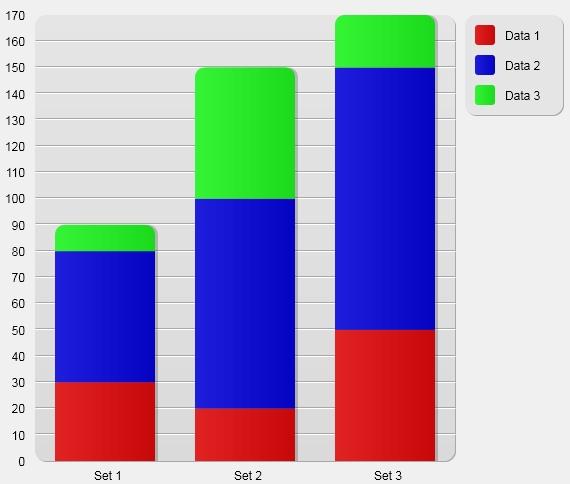
Note: This method will not work on graph type 8, because graph type 8 uses resource images to build the graph.