Tech Tip: Utility method for searching within an object array to find a value
PRODUCT: 4D | VERSION: 15 | PLATFORM: Mac & Win
Published On: October 5, 2015
Below is an utility method for searching within an object array to find a value. The element where the value is found is returned.
//Method Name: UTIL_FIND_IN_OBJECT_ARRAY //$1 - Pointer to object array to search for //$2 - Pointer to value to look for //$3 - Optional, element to start looking for C_POINTER($1;$arrObj;$3;$valToFind) C_TEXT($2;$propertyName) C_LONGINT($4;$start) C_LONGINT($0;$foundElem) C_OBJECT($ob) C_LONGINT($i) C_TEXT($val) C_BOOLEAN($found) If(Count parameters>=2) $arrObj:=$1 $propertyName:=$2 $valToFind:=$3 If (Count parameters>=4) $start:=$4 Else $start:=1 End if $foundElem:=-1 $found:=False $i:=$start While (Not($found)) & ($i<=Size of array($arrObj->)) $ob:=$arrObj->{$i} $val:=String(OB Get($ob;$propertyName)) If ($val=String($valToFind->)) $found:=True $foundElem:=$i End if $i:=$i+1 End while $0:=$foundElem End if |
Here is an example for searching to following object array ($arrObj) for the value "5":
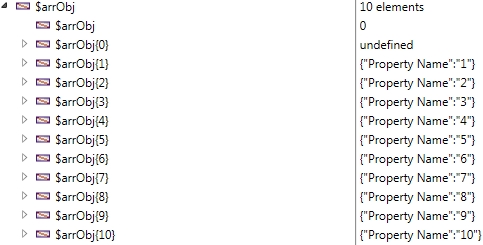
C_TEXT($val;$property) C_LONGINT($elem) $val:="5" $property:="Property Name" $elem:=UTIL_FIND_IN_OBJECT_ARRAY(->$arrObj;$property;->$val) |