Tech Tip: Change selected picture(s) in 4D Write Pro
PRODUCT: 4D | VERSION: 16 | PLATFORM: Mac & Win
Published On: March 9, 2017
4D Write Pro offers the ability to change a picture using the contextual click option "Insert image..." as shown below:
This same functionality can be done programatically with the following method below:
// -------------------------------------------------------------------------------- // Name: WP_CHANGE_SELECTED_PICTURE // Description: Method will input a picture type and modify the picture attribute // of 4D Write Pro Area using Base 64 Encode to the selected picture(s) objects in // the 4D Write Pro UI. // // Input: // $1 (PICTURE) - Picture // $2 (POINTER) - Pointer to the Write Pro Area object // -------------------------------------------------------------------------------- C_PICTURE($1;$pic) C_POINTER($2;$objPtr) C_BLOB($blob;$blob2) C_OBJECT($range) C_TEXT($type;$base64_text) If (Count parameters=2) $pic:=$1 $objPtr:=$2 PICTURE TO BLOB($pic;$blob2;"") COPY BLOB($blob2;$blob;0;0;4) SET BLOB SIZE($blob2;0) Case of : (($blob{0}=255) & ($blob{1}=216)) // JPEG $type:="jpg" : (($blob{0}=66) & ($blob{1}=77)) // BMP $type:="bmp" : (($blob{0}=71) & ($blob{1}=73) & ($blob{2}=70)) // GIF $type:="gif" : (($blob{0}=137) & ($blob{1}=80) & ($blob{2}=78)) // PNG $type:="png" : (($blob{0}=73) & ($blob{1}=73) & ($blob{2}=42)) // TIFF $type:="tif" : (($blob{0}=77)& ($blob{1}=77) & ($blob{2}=0)) // TIFF $type:="tif" : (($blob{0}=60) & ($blob{1}=63) & ($blob{2}=120)) // SVG $type:="svg" : (($blob{0}=54) & ($blob{1}=0)) // PICT $type:="pict" : (($blob{0}=15) & ($blob{1}=154) & ($blob{2}=0)) // 4PCT $type:="4pct" : (($blob{0}=1) & ($blob{1}=0) & ($blob{2}=0)) // EMF $type:="emf" : (($blob{0}=73) & ($blob{1}=73) & ($blob{2}=188)) // WDP $type:="wdp" : (($blob{0}=0) & ($blob{1}=0) & ($blob{2}=1)) // ICON $type:="ico" Else $type:="" // Not determined End case If ($type#"") PICTURE TO BLOB($pic;$blob;"."+$type) BASE64 ENCODE($blob;$base64_text) $base64_text:=Replace string($base64_text;Char(Carriage return);"") $base64_text:=Replace string($base64_text;Char(Line feed);"") $text_set_attr:="data:image/"+$type+";base64,"+$base64_text $range:=WP Get selection($objPtr->) WP SET ATTRIBUTES($range;wk image;$text_set_attr) End if End if |
Here is a sample Write Pro area that contains 3 pictures:
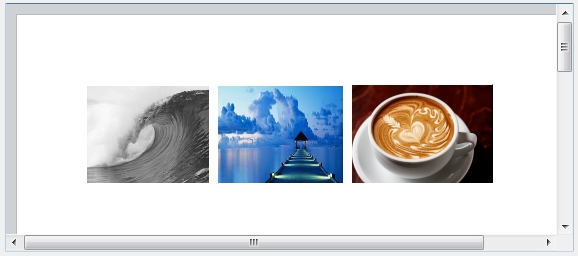
Example #1 Change a selected picture.
This example will select one of the three pictures and change it accordingly to the method. The middle one will be selected.
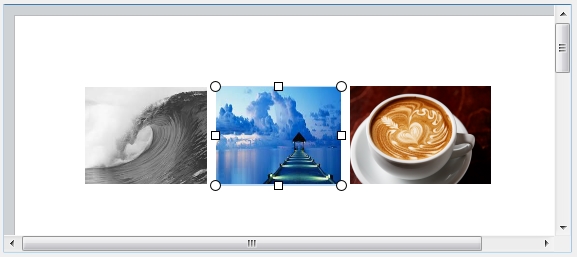
The following code below is executed in a button where it will select a picture from the dialog and replace the current picture:
C_PICTURE($pic) READ PICTURE FILE("";$pic) WP_CHANGE_SELECTED_PICTURE($pic;->WriteProArea) |
Result of the changed picture:
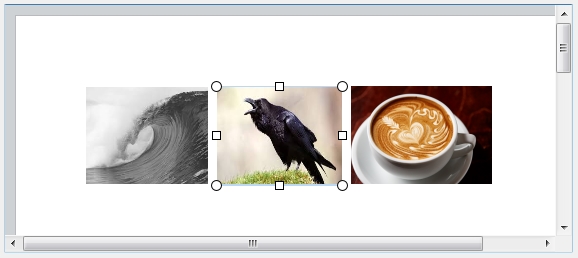
Note: This can also be done with a Picure record as a parameter.
// Assume that a picture was queried in a table WP_CHANGE_SELECTED_PICTURE([Table_1]Field_Picture;->WriteProArea) |
Example #2 Change a selection of pictures:
This example will select two of the three pictures and change it accordingly to the method. The middle and third pictures will be selected.
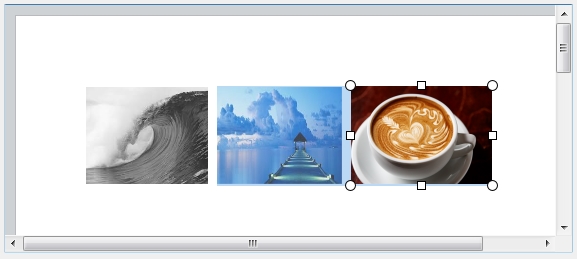
Running the same codes above can have the same effect with the selected pictures:
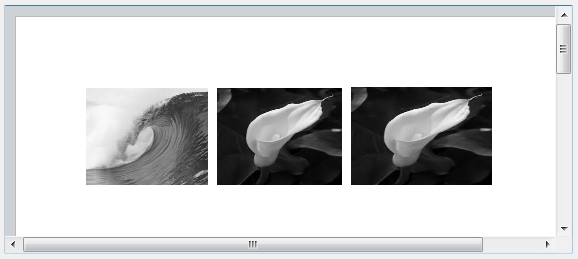
See Also: