Tech Tip: Utility Methods to Round Down and Round Up
PRODUCT: 4D | VERSION: 15.x | PLATFORM: Mac & Win
Published On: December 16, 2016
Below is a utility method to round down and another utility method to round up.
// Util_Floor // Rounds down a number at the number // of decimal places specified // // Parameters: // $1 - Number to be rounded down // $2 - Number of decimal places used for rounding // $0 - Number rounded down to the number of decimal places specified C_REAL($1;$in_r) C_LONGINT($2;$places_l) C_REAL($0;$out_l) $in_r:=$1 $places_l:=$2 $out_r:=Round($in_r;$places_l) If ($out_r>$in_r) $out_r:=$out_r-(1/(10^$places_l)) End if $0:=$out_r |
// Util_Ceil // Rounds up a number at the number // of decimal places specified // // Parameters: // $1 - Number to be rounded up // $2 - Number of decimal places used for rounding // $0 - Number rounded up to the number of decimal places specified C_REAL($1;$in_r) C_LONGINT($2;$places_l) C_REAL($0;$out_l) $in_r:=$1 $places_l:=$2 $out_r:=Round($in_r;$places_l) If ($out_r<$in_r) $out_r:=$out_r+(1/(10^$places_l)) End if $0:=$out_r |
Below is an example of using the commands:
C_REAL($floorRes1;$floorRes2) C_REAL($rndRes1;$rndRes2) C_REAL($ceilRes1;$ceilRes2) C_REAL($rndRes3;$rndRes4) $floorRes1:=Util_Floor (5.77;0) $rndRes1:=Round(5.77;0) $floorRes2:=Util_Floor (5.77;1) $rndRes2:=Round(5.77;1) $ceilRes1:=Util_Ceil (5.33;0) $rndRes3:=Round(5.33;0) $ceilRes2:=Util_Ceil (5.33;1) $rndRes4:=Round(5.33;1) |
Below are the results, compared to a typical round which will round to the nearest decimal.
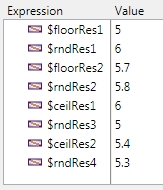