Tech Tip: Finding number of occurrences for distinct attribute values
PRODUCT: 4D | VERSION: 16 | PLATFORM: Mac & Win
Published On: February 23, 2017
The command DISTINCT ATTRIBUTE VALUES can display results from a attribute requested in a selection of field object records. An extension to this command is to find number ocurrances for each found with this method:
// -------------------------------------------------------------------------------- // Name: DISTINCT_ATTR_VALUES_COUNT // Description: Method will look at the selected field object records and extract // the distinct values of a property and number of occurrances. // Input: // $1 (POINTER) - Pointer to the field object object // $2 (TEXT) - Attribute name to find distinct values // $3 (POINTER) - Pointer to an array of disinct values // $4 (POINTER) - Pointer to an array of disinct values occurrances // Output: // $0 (LONGINT) - Error status // - 0: Success // - 1: Wrong array type for distinct values // - 2: Object type undefined in data // -------------------------------------------------------------------------------- C_POINTER($1;$fieldObj) C_TEXT($2;$objName) C_POINTER($3;$arrAttrValues) C_POINTER($4;$arrAttrValuesCnt) C_POINTER($tablePtr) C_LONGINT($type;$pos;$i) If (Count parameters=4) $fieldObj:=$1 $objName:=$2 $arrAttrValues:=$3 $arrAttrValuesCnt:=$4 $tablePtr:=Table(Table($fieldObj)) $fieldPtr:=Field(Table($fieldObj);Field($fieldObj)) // Figure out the type because we don't know the type $type:=OB Get type($fieldPtr->;$objName) If (Type($arrAttrValues->)=Text array) | (Type($arrAttrValues->)=LongInt array) | (Type($arrAttrValues->)=Boolean array) If (($type=Is text) | ($type=Is real) | ($type=Is Boolean)) DISTINCT ATTRIBUTE VALUES($fieldPtr->;$objName;$arrAttrValues->) CLEAR VARIABLE($arrAttrValuesCnt->) INSERT IN ARRAY($arrAttrValuesCnt->;1;Size of array($arrAttrValues->)) For ($i;1;Records in selection($tablePtr->)) If (OB Is defined($fieldPtr->;$objName)) $pos:=Find in array($arrAttrValues->;OB Get($fieldPtr->;$objName)) If ($pos>0) $arrAttrValuesCnt->{$pos}:=$arrAttrValuesCnt->{$pos}+1 End if End if NEXT RECORD($tablePtr->) End for Else $0:=2 // Unknown object type End if Else $0:=1 // Wrong array type End if $0:=0 // Success End if |
Example #1: Finding occurances of age:
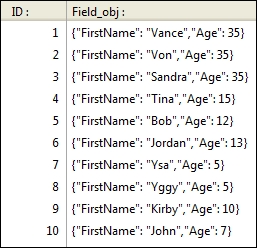
ALL RECORDS([Table_1]) C_LONGINT($err) ARRAY LONGINT(distValues;0) ARRAY LONGINT(distValuesCnt;0) $err:=DISTINCT_ATTR_VALUES_COUNT (->[Table_1]Field_obj;"Age";->distValues;->distValuesCnt) |
Result:
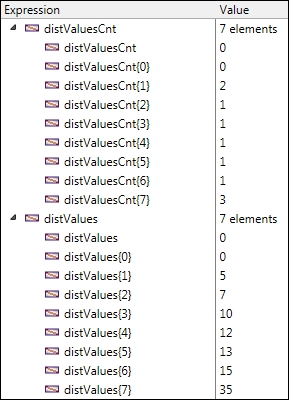
Example #2: Finding occurances of color:
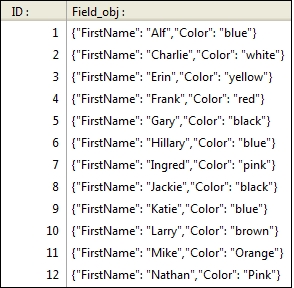
ALL RECORDS([Table_2]) C_LONGINT($err) ARRAY TEXT(distValues;0) ARRAY LONGINT(distValuesCnt;0) $err:=DISTINCT_ATTR_VALUES_COUNT (->[Table_2]Field_obj;"Color";->distValues;->distValuesCnt) |
Result:
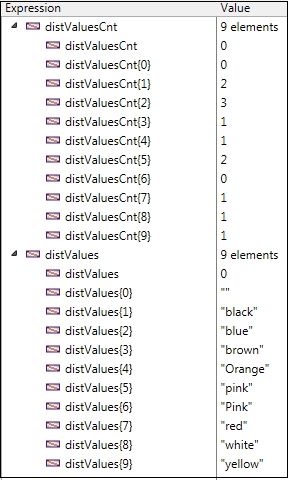
Example #3: Finding occurances of voted:
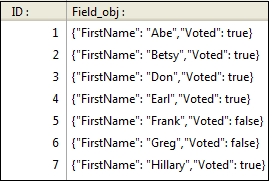
ALL RECORDS([Table_3]) C_LONGINT($err) ARRAY BOOLEAN(distValues;0) ARRAY LONGINT(distValuesCnt;0) $err:=DISTINCT_ATTR_VALUES_COUNT (->[Table_3]Field_obj;"Voted";->distValues;->distValuesCnt) |
Result:
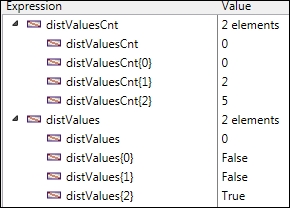
Example #4: Finding DOB
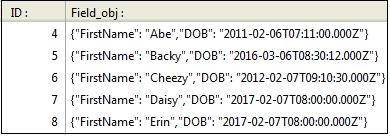
ALL RECORDS([Table_4]) C_LONGINT($err) ARRAY TEXT(distValues;0) ARRAY LONGINT(distValuesCnt;0) $err:=DISTINCT_ATTR_VALUES_COUNT (->[Table_4]Field_obj;"DOB";->distValues;->distValuesCnt) |
Result:
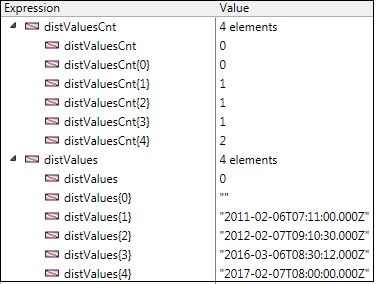
See Also: