Tech Tip: Utility Method to Sort Text Array by String Length
PRODUCT: 4D | VERSION: 15.x | PLATFORM: Mac & Win
Published On: May 19, 2017
Below is a utility method that will take in two pointers to text arrays as a source and destination to sort by string length. An optional parameter can be added to sort in descending order or ascending order. The default will sort in ascending order.
// Util_Array_Text_Length_Sort // // Description: // Takes in a source text array and sorts it in an // output array by length of the strings. // // Parameters: // $1 - Pointer to Source Text Array to be sorted // $2 - Pointer to Destination Text Array to receive sorted result array // $3 - Optional operator selector. // 1 - For Ascending order (default) // -1 - For Descending order // C_POINTER($1) C_POINTER($inArrPtr_p) C_POINTER($2) C_POINTER($outArrPtr_p) C_LONGINT($3) C_LONGINT($orderSelector_l) If (Count parameters>1) C_LONGINT($arrCount_l) C_LONGINT($count_l) ARRAY LONGINT($arrLen_al;0) $inArrPtr_p:=$1 $arrCount_l:=Size of array($inArrPtr_p->) $outArrPtr_p:=$2 If (Count parameters>1) $orderSelector_l:=$3 Else $orderSelector_l:=1 End if For ($count_l;1;$arrCount_l) APPEND TO ARRAY($arrLen_al;Length($inArrPtr_p->{$count_l})) End for COPY ARRAY($inArrPtr_p->;$outArrPtr_p->) If($orderSelector_l=-1) MULTI SORT ARRAY($arrLen_al;<;$outArrPtr_p->) Else MULTI SORT ARRAY($arrLen_al;>;$outArrPtr_p->) End if End if |
Below is an example of using the method:
ARRAY TEXT($sourceArr_at;0) APPEND TO ARRAY($sourceArr_at;"aa") APPEND TO ARRAY($sourceArr_at;"a") APPEND TO ARRAY($sourceArr_at;"aaaa") APPEND TO ARRAY($sourceArr_at;"aaa") APPEND TO ARRAY($sourceArr_at;"aa") APPEND TO ARRAY($sourceArr_at;"aaaaaa") ARRAY TEXT($resultsAscending_at;0) ARRAY TEXT($resultsDescending_at;0) Util_Array_Text_Length_Sort (->$sourceArr_at;->$resultsAscending_at) Util_Array_Text_Length_Sort (->$sourceArr_at;->$resultsDescending_at;-1) TRACE |
Taking a look at the results in the debugger shows:
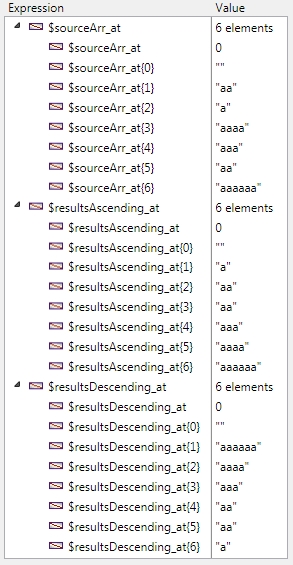