Tech Tip: What is a Range Checking Error
PRODUCT: 4D | VERSION: 17 | PLATFORM: Mac & Win
Published On: August 14, 2019
Range checking (enabled by default) provides additional analysis of the code at runtime. Range checking evaluates the status of objects in the database at the time of code execution. Range checking messages do not appear in interpreted context, they only appear when your database is running in the compiled context.
Range Checking errors are not typically caught by an error handler that is installed by ON ERR CALL. Range checking errors can be avoided by using Defensive Programming techniques. Here are some examples of Range Checking errors and the bad code that caused them:
-20001: Array capacity exceeded
MyArray is three-element array when the statement is executed.This message appears if you try to access beyond element 3 in the array.
ARRAY TEXT(MyArray;3) MyArray{1}:="this is OK" MyArray{2}:="this is OK" MyArray{3}:="this is OK" MyArray{4}:="this produces an out of range runtime error" |
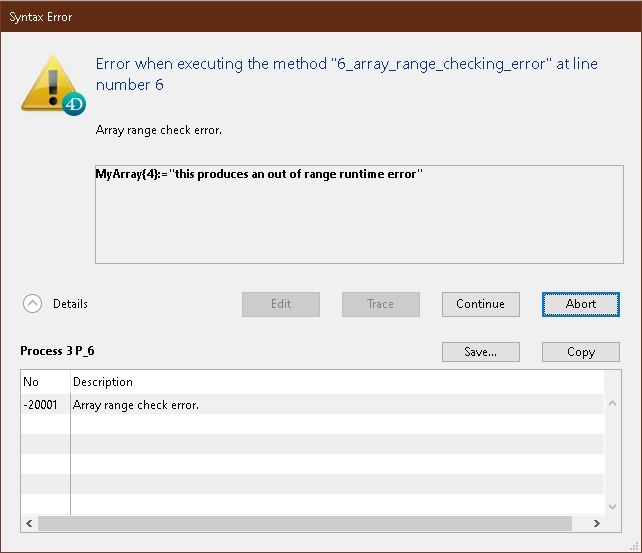
-20002: The parameter cannot be passed.
Using the $2 local variable when the parameter has not been passed to the current method.ALERT($2) |
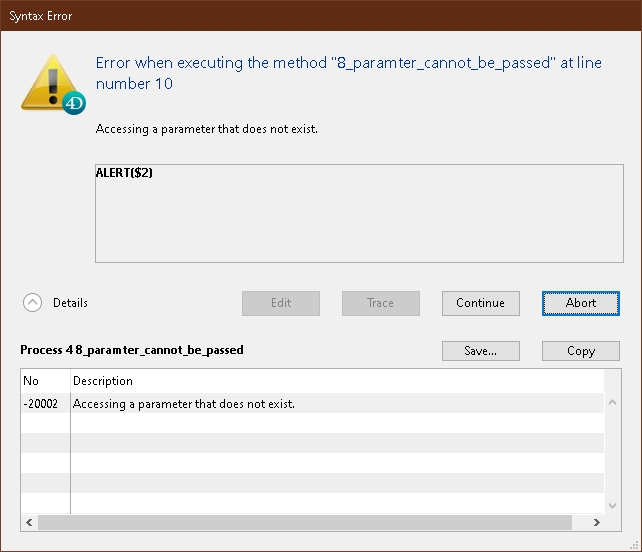
-20003: The pointer is not correctly initialized.
if the pointer has not yet been initialized (never had anything assigned to it).C_POINTER(MyPointer) MyPointer->:=5 |
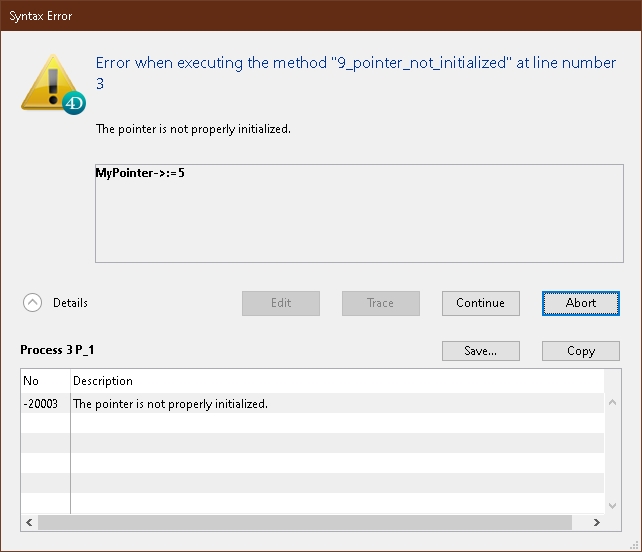
-20004: The destination string is smaller than the source.
error -20004 is linked to C_STRING variables and their lengths. C_STRING variables have been deprecated.-20005: Incorrect usage of a pointer
if Pointer does not point to a number.C_TEXT(StringVar;Character;testVar) C_POINTER(Pointer) StringVar:="This is a test" testVar:="test" Pointer:=->testVar Character:=StringVar[[Pointer->]] |
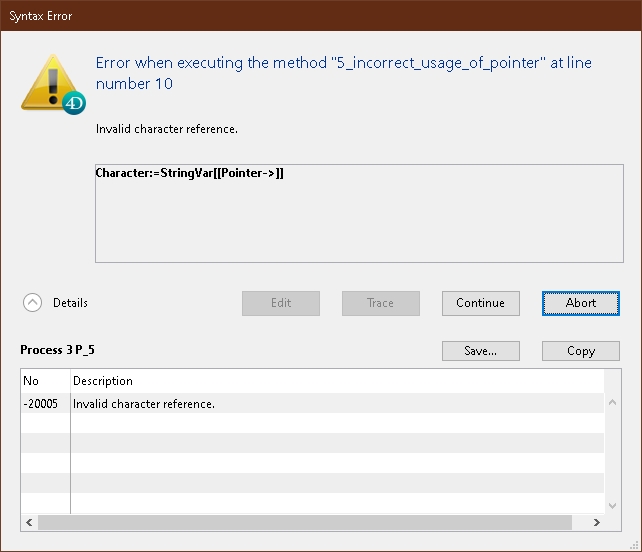
-20005: The string index isn’t valid (too large or negative).
C_TEXT(MyString;MyString2) MyString:="test" i:=30 MyString2:=MyString[[i]] |
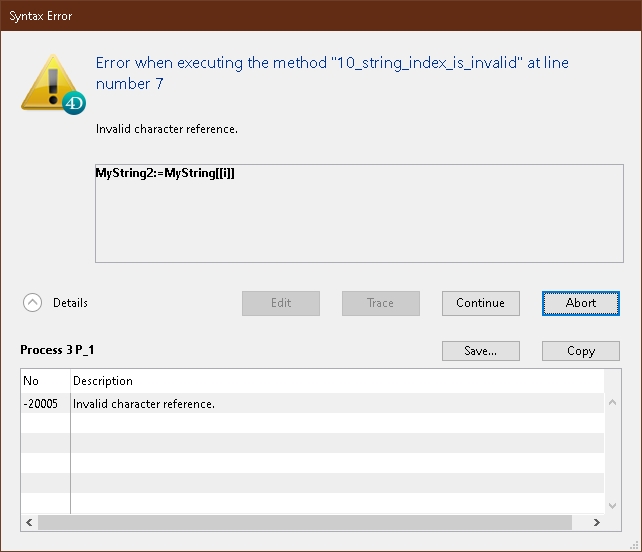
-20006: The passed string in the parameter is empty or not initialized.
C_TEXT(MyString;MyString2) MyString:="test" i:=2 MyString[[i]]:="" |
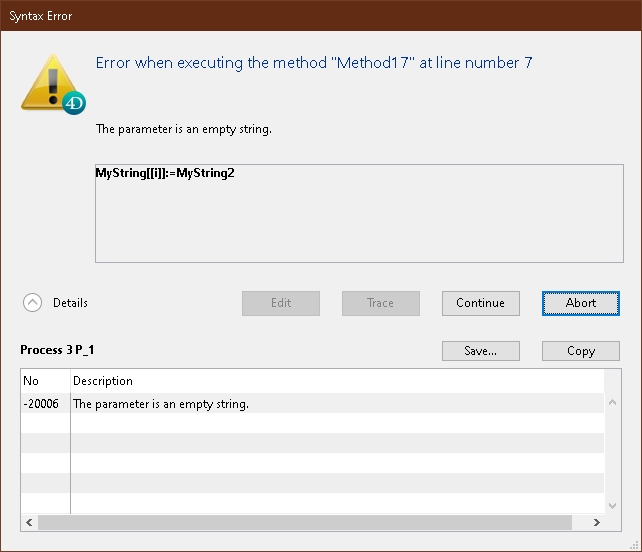
-20007: Tried to retype by using a pointer
if Pointer points to field of type Integer.
C_BOOLEAN(BoolVar) C_POINTER(Pointer) C_LONGINT(long) long:=12 Pointer:=->long BoolVar:=Pointer-> |
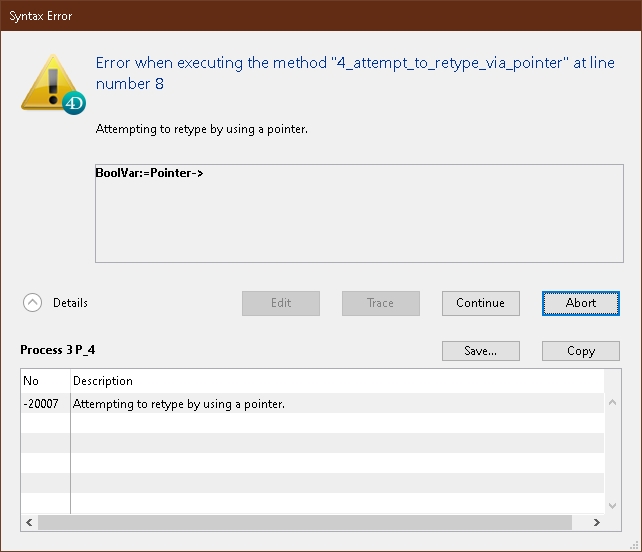
-20008: Invalid parameters in an EXECUTE
if Variable does not appear explicitly in the database.EXECUTE FORMULA("Method(Variable)") |
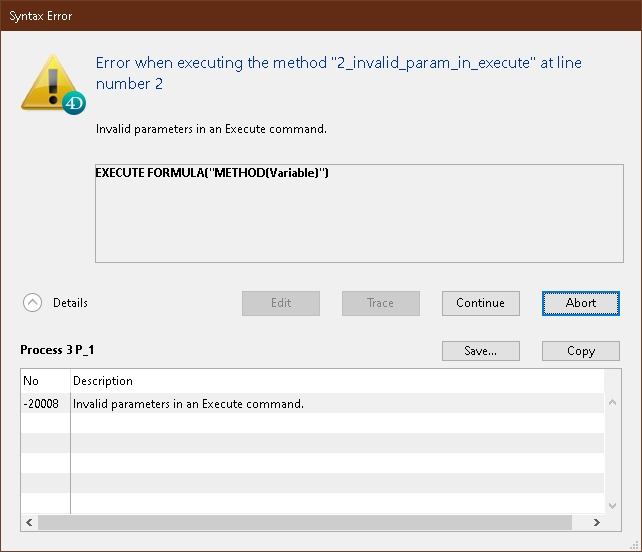
-20009: Pointer to an unknown variable
if the pointer has not yet been initialized (never had anything assigned to it).-20010: Bad usage of a pointer or pointer to an unknown variable
if the pointer has not yet been initialized (never had anything assigned to it).-20011: Divide by zero
Attempting to perform on division on zero produces a range checking error:Var1:=0 Var2:=2 Var3:=Var2%Var1 |
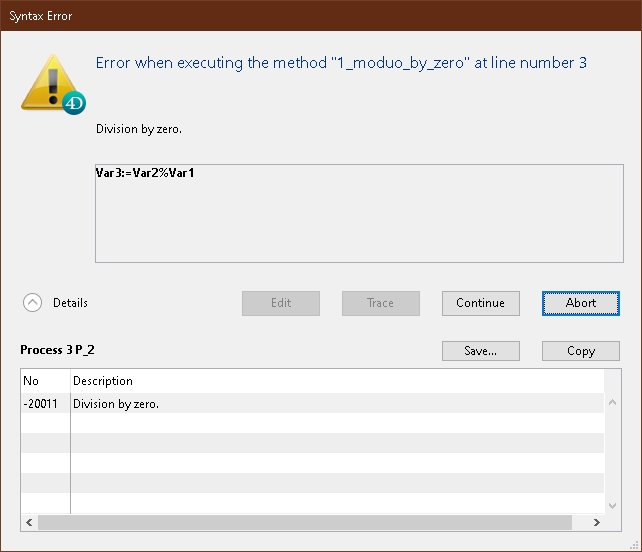
-20012: Modulo by zero
Attempting to perform on modulo on zero produces a range checking error-20013: The result is out of range
Typically range checking errors can be avoided by using Defensive Programming techniques.
See Also: