Transactions are useful when designing forms for a database application. A transaction can be understood as a series of related modifications to a database within a process. Transactions can be nested, so that multiple transaction levels can occur at the same time in a process.
In 4D there are several commands for transactions which are Active transaction, In transaction, and Transaction level which provide basic information on transactions and for using transactions START TRANSACTION, CANCEL TRANSACTION, SUSPEND TRANSACTION, RESUME TRANSACTION, and VALIDATE TRANSACTION.
Transactions are started with the START TRANSACTION command, and will remain active until cancelled, suspended, or validated. Validating a transaction with the VALIDATE TRANSACTION command will commit the changes made during the transaction to the database. Using the CANCEL TRANSACTION command will cancel any changes made and they will not be commited to the database.
The most common example for understanding suspension of transactions, and how they work, comes when considering an invoicing application.
Imagine using an invoicing system and creating a new invoice for a customer.
During the creation of the invoice, it is noticed that the details for the customer are incorrect, and so they need to be updated! Thankfully the developer has added a "Modify Customer" button on the invoice creation form, so clicking on this brings up the customer record to be modified.
After modifying the customer information, and saving it to the database, the application returns to the invoice form but now the situation has changed and the invoice no longer needs to be filled. Maybe the customer changed their mind, or the order has to be delayed, for whatever reason the invoice is cancelled.
After hitting cancel, the desired effect would be for the address change to be saved in the database, even though the invoice was not saved in the end, because the update of the customer's address is valid.
This is accomplished with transactions.
When loading the invoice from the dashboard, the invoice form is opened. Looking at the form method, the form event On Load starts a transaction.
// invoice form method Case of : (Form event=On Load) START TRANSACTION // load records as needed, other initializations End case |
The Modify Customer button takes the updated address and saves it to the database by suspending the current transaction (the invoice) starting a new transaction (the address update) and then saving that in the database and validating it, before resuming the transaction again for the invoice.
// modify customer button method C_BOOLEAN($wasInTransaction) $wasInTransaction:=False If (In transaction) SUSPEND TRANSACTION $wasInTransaction:=True End if QUERY([Customer];[Customer]name=customer_name) If (Records in selection([Customer])=1) START TRANSACTION [Customer]address:=customer_address SAVE RECORD([Customer]) VALIDATE TRANSACTION Else ALERT("Multiple customers of same name found") End if If ($wasInTransaction) RESUME TRANSACTION End if |
The Cancel button simply cancels the transaction and has a standard action as cancel.
// code for cancel button method CANCEL TRANSACTION |
The Save Invoice button saves the invoice to the database and validates the transaction.
// save invoice button method CREATE RECORD([Invoice]) [Invoice]date:=vDate [Invoice]desc:=desc_t [Invoice]price:=price_r QUERY([Customer];[Customer]name=customer_name) If (Records in selection([Customer])=1) [Invoice]cus_id:=[Customer]ID SAVE RECORD([Invoice]) VALIDATE TRANSACTION Else ALERT("Multiple customers found with that name") End if |
Graphic representation of the above:
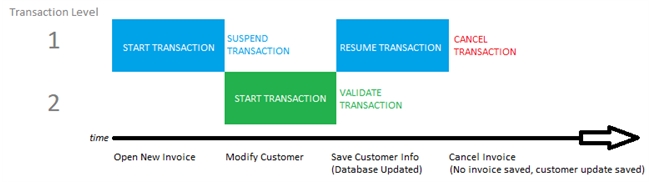