Tech Tip: Generate and verify a hash collection
PRODUCT: 4D | VERSION: 16R6 | PLATFORM: Mac & Win
Published On: March 2, 2018
In v16R6, the Collection type functionality is growing through 4D versions. Data can be easily displayed in different types through a Collection. A Collection can easily be used for transmission of data. One way is to hash the data and and have it verified with the original data. The following utility method can generate a hash of a collection and verify the hash for accuracy:
// ---------------------------------------------------------------------- // Name: GENERATE_CHECK_HASH_COLLECTION // Description: Method will take a collection and create a hash // collection as well as verifying a hashed collection. // // Parameters: // $1 (COLLECTION) - Collection to hash // $2 (COLLECTION) - Hash collection to verify original collection // Output: // $0 (COLLECTION) // E.g. [0]:0 // Successful hash generated for original collection // [0]: // No Hash collection created // [0]:N // Fail where 'N' is number times hash is inaccurate // [0]:-1 // Invalid number of parameters // ---------------------------------------------------------------------- C_COLLECTION($1;$origCol;$collect) C_COLLECTION($2;$hashCol) C_COLLECTION($0) C_LONGINT($i;$count) C_TEXT($text) If (Count parameters>0) $origCol:=$1 $collect:=New collection If (Count parameters=2) $hashCol:=$2 $count:=0 End if For ($i;0;$origCol.length-1) If ((Value type($origCol[$i])=Is object) | (Value type($origCol[$i])= \ Is collection) | (Value type($origCol[$i])=Is pointer)) $text:=Generate digest(JSON Stringify($origCol[$i]);SHA512 digest) Else $text:=Generate digest(String($origCol[$i]);SHA512 digest) End if If (Count parameters=1) $collect.push($text) Else If ($text#$hashCol[$i]) $count:=$count+1 End if End if End for If (Count parameters=1) $0:=$collect Else $0:=$collect.push($count) End if Else $0:=New collection(-1) End if |
Here is an example of creating a Collection hash:
C_COLLECTION($col;$colHash) $col:=New collection("banana";"cherry";"orange";"apple";"cherry";"orange") $colHash:=GENERATE_HASH_COLLECTION ($col) |
The Collection $colHash will look like the following:
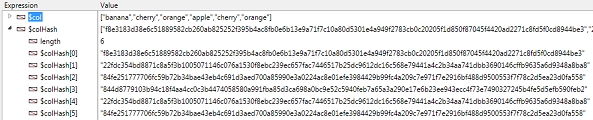
Using the same GENERATE_CHECK_HASH_COLLECTION to verify:
C_COLLECTION($colStatus) $colStatus:=GENERATE_CHECK_HASH_COLLECTION ($col;$colHash) // [0] - 0 (Success) |
Here is another example with different types:
C_COLLECTION($col) C_OBJECT($obj) OB SET($obj;"prop";1) $col:=New collection(1234;Current time;Current date;$obj) $colHash:=GENERATE_HASH_COLLECTION ($col) |
