Tech Tip: Extracting pages from a WP document into a new WP document
PRODUCT: 4D | VERSION: 17 | PLATFORM: Mac & Win
Published On: May 15, 2019
Here is a utilty method to extract pages in a Write Pro document and copy it to a new Write Pro document programatically:
// ---------------------------------------------------------------------- // Name: EXTRACT_PAGES_TO_NEW_WP_AREA // Description: Method will extract the pages from the Write Pro area // and create a new Write Pro Area object. // // Parameters: // $1 (POINTER) - Pointer to the Write Pro Area // $2 (LONGINT) - First page to extract // $3 (LONGINT) - Last page to extract // // Output: // $0 (OBJECT) - New Write Pro Object // ---------------------------------------------------------------------- C_POINTER($1;$writeProObj) C_LONGINT($2;$pageChoice;$nbPages;$page;$tableName;$fieldName) C_LONGINT($3;$pageChoice2) C_LONGINT($start_pos;$end_pos) C_OBJECT($0;$range;$wpObj;$body;$info;$paragraph) C_TEXT($varName) C_BOOLEAN($start_read) C_COLLECTION($_paragraphs) If (Count parameters>=2) $writeProObj:=$1 $pageChoice:=$2 $pageChoice2:=0 If (Count parameters=3) $pageChoice2:=$3 End if $start_read:=False $nbPages:=WP Get page count($writeProObj->) $body:=WP Get body($writeProObj->) $_paragraphs:=WP Get elements($body;wk type paragraph) $start_read:=False For each ($paragraph;$_paragraphs) $info:=WP Get position($paragraph) $page:=OB Get($info;"page") WP SELECT($writeProObj->;$paragraph) RESOLVE POINTER($writeProObj;$varName;$tableName;$fieldName) $range:=WP Get selection(*;$varName) If ($page=$pageChoice) If ($start_read=False) $start_read:=True $start_pos:=$range.start End if $end_pos:=$range.end End if If ($page=$pageChoice2) $end_pos:=$range.end End if End for each $range:=WP Create range($writeProObj->;$start_pos;$end_pos) // Go back to the beginning WP SELECT($writeProObj->;$start_pos;$start_pos) $0:=WP New($range) End if |
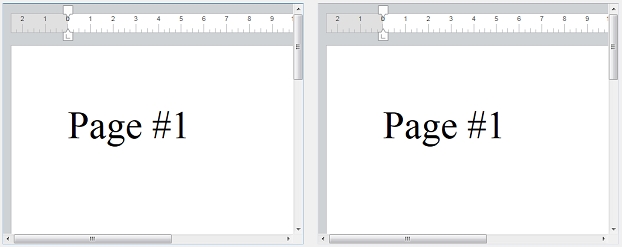
Here is an example of using the method of extracting page 1 and 2:
C_OBJECT($obj) $obj:=EXTRACT_PAGES_TO_NEW_WP_AREA (->WriteProArea1;1;2) WriteProArea2:=$obj |
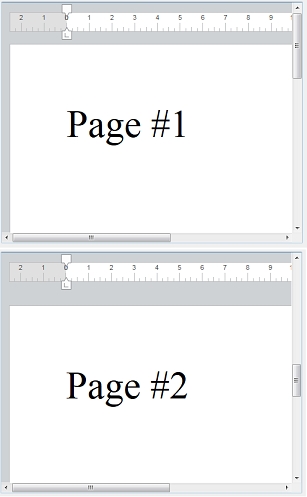