Tech Tip: Utility Method to Get Dataclass from Entity
PRODUCT: 4D | VERSION: 17 | PLATFORM: Mac & Win
Published On: November 29, 2018
This utility method UTIL_Get_Dataclass_From_Entity will take an entity/entity selection as input and search through all tables at that entity's key value to see whether the object contents match the given entity. In order to compare two entities from different tables, the objects are converted to text using JSON Stringify.
// ---------------------------------------------------- // User name (OS): Erick Lui // Date and time: 10/24/18, 09:43:32 // ---------------------------------------------------- // Method: UTIL_Get_Dataclass_From_Entity // Description: // Utility method that returns name of dataClass from given entity/entity selection // // Parameters // $0 - Data class name (TEXT) // $1 - Entity/Entity selection (OBJECT) // ---------------------------------------------------- C_OBJECT($1;$ent1) C_TEXT($0;$dataClassName) C_OBJECT($ent2) C_TEXT($entText1;$entText2;$tableName) C_TEXT($key) If (Count parameters=1) $ent1:=$1 // If input is entity selection, get only first entity If ($ent1.length#Null) $ent1:=$ent1.first() End if // If entity is not empty, convert to JSON string and get key If ($ent1#Null & Not(OB is empty($ent1))) $entText1:=JSON Stringify($ent1.toObject("";dk with primary key)) $key:=$ent1.getKey(dk key as string) // Check all tables at specified key and compare entity contents For each ($tableName;ds) $ent2:=ds[$tableName].get($key) If ($ent2#Null) $entText2:=JSON Stringify($ent2.toObject("";dk with primary key)) // If entity contents match, set output to tableName If ($entText1=$entText2) $dataClassName:=$tableName End if End if End for each End if End if // Return dataClassName if found, otherwise return empty string $0:=$dataClassName |
Here's an example database structure with two tables: one table with a longint primary key and another table with UUID primary key.
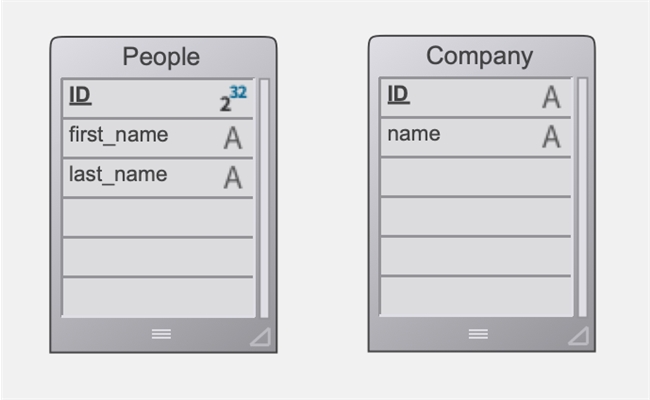
Below is code that calls the method and passes different entities and entity selections to UTIL_Get_Dataclass_From_Entity.
C_OBJECT($entSelection1;$ent1) C_OBJECT($entSelection2;$ent2) C_TEXT($dataClassName1;$dataClassName2;$dataClassName3;$dataClassName4) $entSelection1:=ds.Company.all() $ent1:=$entSelection1.first() $entSelection2:=ds.People.all() $ent2:=$entSelection2.first() // pass Company entity selection as argument $dataClassName1:=UTIL_Get_Dataclass_From_Entity ($entSelection1) // pass Company entity as argument $dataClassName2:=UTIL_Get_Dataclass_From_Entity ($ent1) // pass People entity selection as argument $dataClassName3:=UTIL_Get_Dataclass_From_Entity ($entSelection2) // pass People entity as argument $dataClassName4:=UTIL_Get_Dataclass_From_Entity ($ent2) |
Lastly, the output from the utility method.
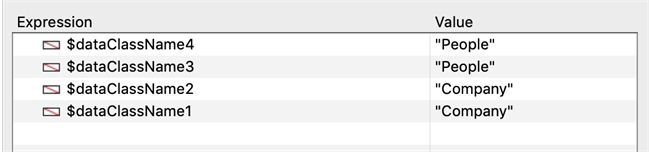