Tech Tip: How to iterate through the properties of an object
PRODUCT: 4D | VERSION: 19 | PLATFORM: Mac & Win
Published On: April 24, 2023
You can use the following loop structure in order to loop through each property of an object:
For each ($property; $object) // insert what you would like to do with each property here End for each |
The following are a couple use cases where this loop structure can be used:
1) Get all properties of an object
getObjectProperties Method
// Get Object Properties Method // gets all properties of an object and returns them as a collection // declare input and output parameters #DECLARE($object : Object)->$propertiesArray : Collection // create collection $propertiesArray:=New collection // get all properties and insert into collection For each ($property; $object) If (Value type($property)=Is text) $propertiesArray.insert($propertiesArray.length+1; $property) End if End for each |
Tester Method
// Get Object Properties Tester Method // tests the getObjectProperties method // declare variables var $contact : Object // create sample object $contact:=New object $contact.firstName:="John" $contact.lastName:="Doe" $contact.phone:=1234567890 $contact.addressLine1:="100 1st St." $contact.addressLine2:="" $contact.city:="New York" $contact.state:="New York" $contact.zipcode:=10001 // get all properties of object $properties:=getObjectProperties($contact) |
Result:
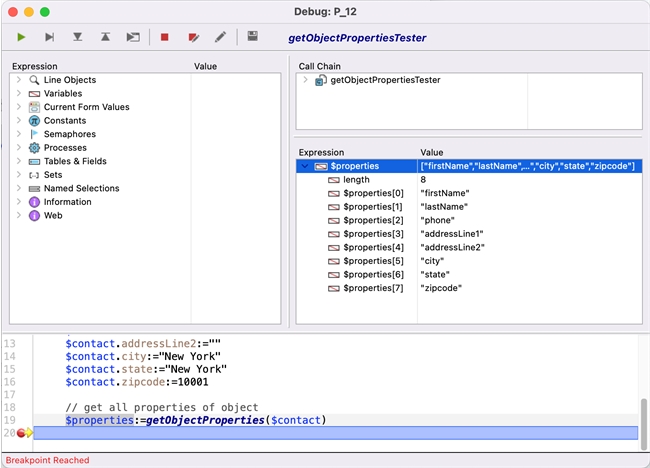
2) Manipulate each property value
Example code snippet
// declare variables var $yearlySales : Object var $property : Text // create sample object $yearlySales:=New object $yearlySales.q1:=25000 $yearlySales.q2:=38000 $yearlySales.q3:=29000 $yearlySales.q4:=33000 // loop through each property and adjust quarterly amounts by $2000 For each ($property; $yearlySales) If (Value type($yearlySales[$property])=Is real) $yearlySales[$property]:=$yearlySales[$property]+2000 End if End for each |