This tech tip was inspired by https://kb.4d.com/assetid=77740, which can be used for binary databases.
Although the system color picker in Windows and MacOS can be invoked via the OPEN COLOR PICKER command, it does not return the value of the RGB color selected by the user. However, a workaround can be used using an input box with the Allow font/color picker property.
3 elements are needed for this implementation:
1. Offscreen Text Input Box
2. Variable to save RGB color value
3. Code to invoke the Color Picker dialog and get a selected color
1. For the first part, add an offscreen Text Input Box to a form. Make sure that it has the Enterable and Allow font/color picker properties enabled and the Multi-style property disabled. An example with coordinates and sizing can be found below:
2. Next, you will need a variable that will hold the RGB color value in the context of the whole form. For demonstration purposes, a process variable color is used, but the value can also be saved inside form data or as a method return value to be used in other code.
3. The Button object method and form method below demonstrate the general logic used for this implementation. The Button object is used to invoke the Color Picker and shift the form object focus to the offscreen Text Input Box. Once the Color Picker dialog is open, the user can select a color. Because the Text Input has the Allow font/color picker property enabled, the selected color in the Color Picker dialog will be applied to the text of the offscreen text input. The text color can then be obtained, using the OBJECT GET RGB COLORS command. The RGB value is then saved into a variable, which can then be applied to other form elements (e.g., a rectangle form element).
// Button Object Method // Opens the Color Picker dialog and sets the color to black // Sets the form element focus to the offscreen text input // open Color Picker + set color to black OPEN COLOR PICKER(0) // "0" parameter will apply to text color in text input box OBJECT SET RGB COLORS(*; "Input1"; "#000000"; "#000000") // set focus to offscreen text input GOTO OBJECT(*; "Input") |
// Form Method // During On Load, declares the color variable // During On After Edit, gets the selected RGB color and applies it to a rectangle form element Case of // declare variable (that holds RGB color value) : (FORM Event.code=On Load) var color : Text // gets the selected color and applies it to a form element // Note: "On After Edit" is invoked every time a color is selected : (FORM Event.code=On After Edit) // grab the color OBJECT GET RGB COLORS(*; "Input"; color) // set the fill color of the rectangle OBJECT SET RGB COLORS(*; "Rectangle"; "#000000"; color) // black border, fill with color from color picker End case |
The result of this implementation is a color-filled rectangle, based on the color selected in the Color Picker:
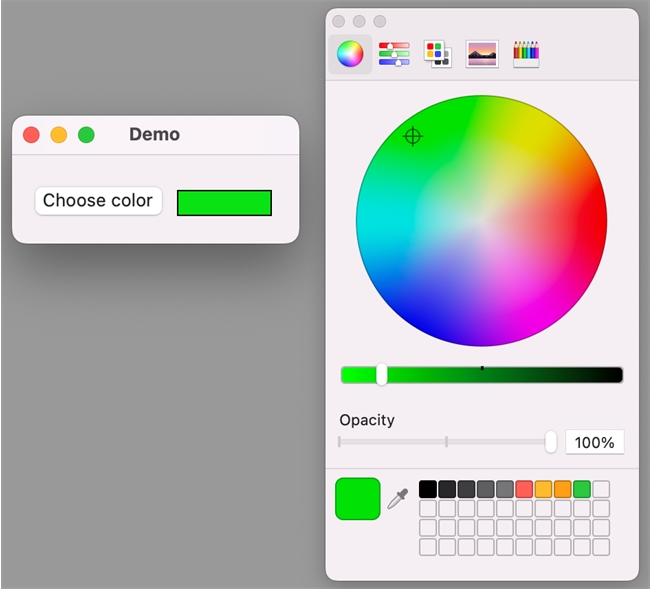
Notes: Unlike the Select RGB Color command, the implementation above allows for the selected RGB value to be returned while the Color Picker dialog is still open. Moreover, an Integer value can be used in place of a text value when working with the OBJECT SET RGB COLORS and OBJECT SET RGB COLORS commands.